Real time data processing with Apache Beam and Java
Unleashing the Potential of Real-Time Analytics with Apache Beam and Java
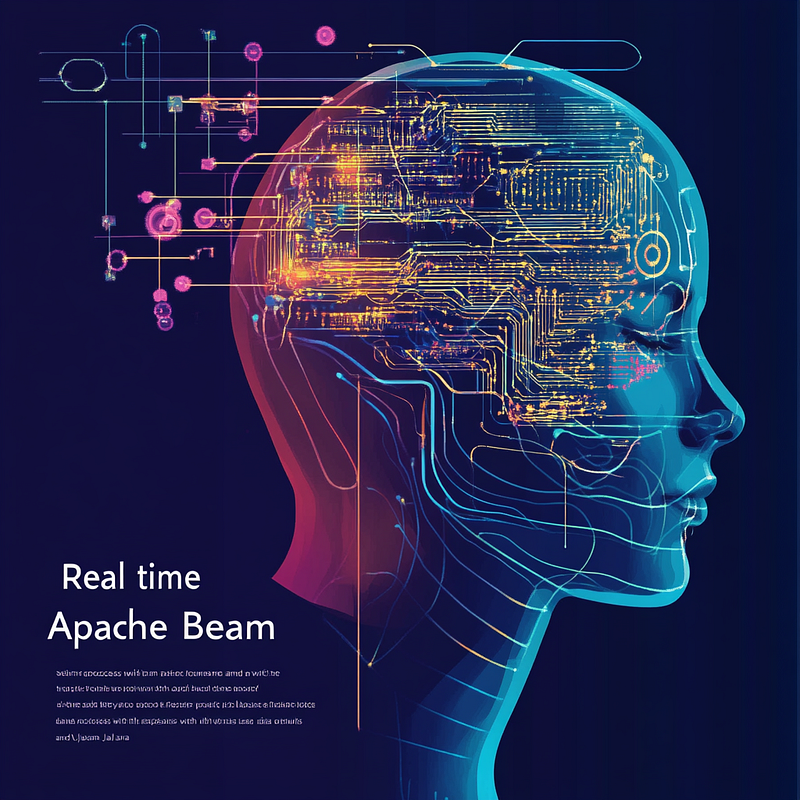
Introduction
Today world is fast — paced digital landscape, real-time data processing has become a crucial requirement for many businesses. The read — world case scenarios for employ real — time data processing are analyzing user behaviour, monitoring IoT devices or detecting fradulent activities. This is where Apache Beam comes into play.
Apache Beam is an open — source, unified programming model for building batch and streaming data processing pipelines. Apache Beam enables developers creating data processing workflows in powerfull and flexible way.
In this article, we will dive into Apache Beam and explore how it can be used with Java to build efficient and scalable real-time data processing pipelines. This article cover the core concepts of Apache Beam as well as its key components, and real — wolrd use case scenarios. I will show Java code to illustrate how to implment Apache Beam pipelines in practice. In the end of this article, you will have a understanding of how to use Apache Beam with Java.
What is Apache Beam ?
As discussed per introduction section Apache Beam is an open — source unified programming model for buuilding data processing pipelines for both batch and streaming data. By providing high — level abstraction layer it allows developers to focus on the business logic of their data processing tasks, while the underlying execution engine handles the low — level details of distrubuted computing.
One of main benefits of Apache Beam it its portability. Pipelines can be executed on various backends, including Apache Flink, Apache Spark, and Google Cloud Dataflow, without requiring sifnificant modifications to the code. The portability make give option to developers to choose the most suitable execution engine based on their specific requirements and infastructure setup.
Apache Beam follows a declarative programming model, so developers dfine the data processing workflow using a set of transformations and operations. The transformation applied to data collections called PCollections, which represent a potentially unbounded dataset. By rich set of built-in transformations, such as ParDo (parallel do), GroupByKey, Combine, and Flatten, that allow developer to perform common data processing tasks efficiently.
How Apache Beam Works
Let’s break down its core components and concepts to better understanding how Apache Beam works.
- Pipeline: A pipeline represents the entire data processing workflow in Apache Beam. The pipeline encapsulates the series of transformations and operations that applied to the input data to produce the desired output. Below is an example of creating a pipeline in Java
Pipeline pipeline = Pipeline.create(options);
2. PCollection: This is abstraction of a potentially unbounded dataset in Apache Beam. It represnt a collection of data elements that can be processed in parallel way. PCollections are immutable, meaning they cannot be modified once created. Below example of creating PCollection from a list of integers.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
PCollection<Integer> pCollection = pipeline.apply(Createo.of(numbers))
3. Transformations: This is building block of a Beam pipeline. It is defines the operations that are applient to the input PCollection to produce one or more output PCollections. Apache Beam has a lot of built-in transformation. Below is example of transofrmation
PCollection<Integer> squaredNumbers = pCollection.apply(ParDo.of(new DoFn<Integer, Integer>() {
@ProcessElement
public void processElement(ProcessContext c) {
int number = c.element();
c.output(number * number);
}
}));
4. ParDo: It is tranformation that applies a user — defined function to each element in the input PCollection. It enables developers to perform custom processing logic on individual elements in parallel. In the code above ParDo used to square each element of the input PCollection.
5. Windowing: Windowing is a concept in Apache Beam that allows developers to group elements based on specific criteria. It is useful concept in situations where data arrives continiously and processing need to be peformed over a finite subset of the data. Below is an example of applying a fixed — time windowing strategy to a PCollection
PCollection<Integer> windowedData = pCollection.apply(Window.<Integer>into(FixedWindows.of(Duration.standardMinutes(5))));
6. Triggers: They define conditions under which the data processing pipeline should produce output, such as when a certian number of element have been processed or a speccific time interval has elapsed. Below is example of applying a trigger that fires when the window is closed:
PCollection<Integer> triggeredData = windowedData.apply(Window.<Integer>triggering(AfterWatermark.pastEndOfWindow()));
7. Watermarks: It is mechanism to track the progress of time in a streaming pipeline. They represent a point in the data stream where all data up to that point has been processed. Watermakrs are used to determine when windows can be closed and results can be emitted.
Real-World Use Case Scenarios
Now since we get understanding of core concepts of Apache Beam and its core component, let’s elaborate some real — world use case scenarios where Apache Beam and Java can be applied for real — time data processing.
- Real — time user behaviour analysis: Let’s imagine an e — commerce platform that wnats to analyze user behavior in real time to personalize recommendations and improve user experince. With leveraging Apache Beam and Java, you can build a streaming pipeline that ingests user clickstream data, applies transofrmation to extract relevant features, and perform real — time data processing. In order to implement this we should use windowing and triggers, so we can process user data in near real-time and generate actionable insights. Below code snippet that demonstrates how to build streaming pipeline to analuyze user click events:
PCollection<KV<String, Long>> userClickCounts = pipeline
.apply("ReadClickEvents", PubsubIO.readStrings().fromTopic("click_events"))
.apply("ParseJSON", ParDo.of(new ParseClickEventFn()))
.apply("ExtractUserID", ParDo.of(new ExtractUserIDFn()))
.apply("WindowByUser", Window.into(FixedWindows.of(Duration.standardMinutes(5))))
.apply("CountUserClicks", Count.perElement());
The pipeline reads user click events from a Pub/Sub topic, parses the JSON data, extracts the user ID, applies a fixed time window of 5 minutes, and counts the number of clicks per user within each window.
2. IoT Device Monitoring: Real — time data processing is vital for monitoring and managing connected devices. Apache Beam and Java can be used to build a pipeline that ingests sensor data from IoT devices, applies tranformation to detect anomalies or patters, and triggers appropriate actions. Below a code snippet that demonstrate how it can look like in Java
PCollection<KV<String, Double>> anomalies = pipeline
.apply("ReadSensorData", PubsubIO.readStrings().fromTopic("sensor_data"))
.apply("ParseJSON", ParDo.of(new ParseSensorDataFn()))
.apply("DetectAnomalies", ParDo.of(new AnomalyDetectionFn()))
.apply("FilterAnomalies", Filter.by(new AnomalyFilterFn()));
3. Fraud Detection: it is very required mechanism in industries such as banking and insurance. Apache Beam and Java can be employed to build a real — time fraud detection system that analyzes transactional data and identifies suspicious patterns.
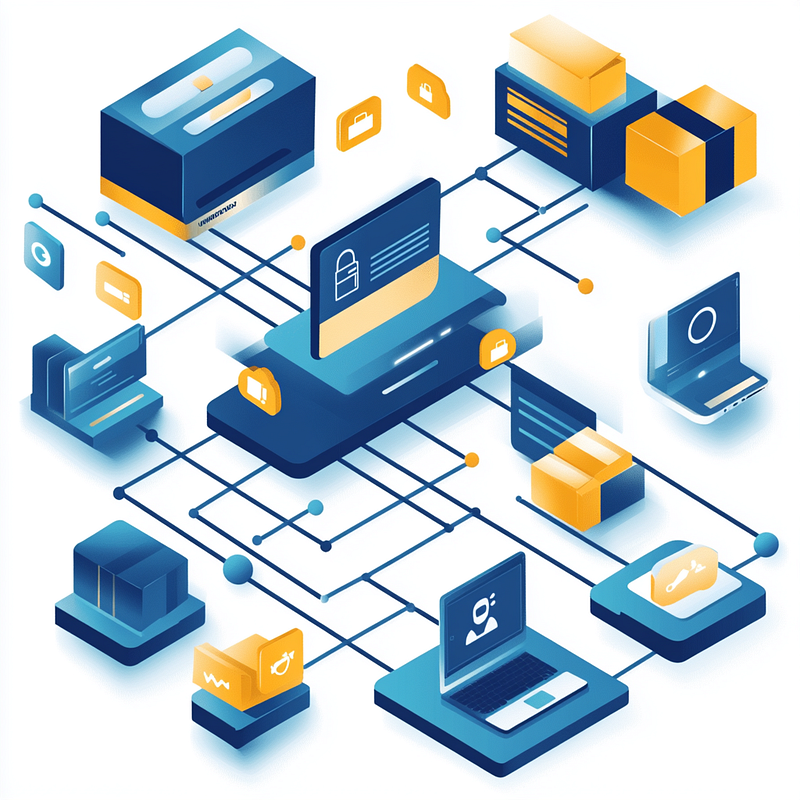
Below a code snippet:
PCollection<Transaction> fraudulentTransactions = pipeline
.apply("ReadTransactions", PubsubIO.readAvros(Transaction.class).fromTopic("transactions"))
.apply("ExtractFeatures", ParDo.of(new ExtractFeaturesFn()))
.apply("DetectFraud", ParDo.of(new FraudDetectionFn()))
.apply("FilterFraudulent", Filter.by(new FraudulentTransactionFilterFn()));
In this example, the pipeline reads transaction data from a Pub/Sub topic using Avro format, extract relevant features, applies a fraud detection function to identify suspicious transactions, and filters out the fraudlent transactions for furtther action.
Conclusion
Apache Beam is go-to tools for building real — time data processing pipelines using Java. With leveraging unified programming model, rich set of transformations, and portability across different execution engines make it a good choice for developers working on data — intensive applications.
In this article, we explored the core concepts of Apache Beam, its core components, and how it can be used with Java to solve real — time data processing challenges. This article illustrate examples of to implement basic pipelines. We also discussed real — world use case scenarios, such as real — time user behaviour analysis, IoT device monitoring and fraud detection, in all these cases Apache Beam and Java can be applied effectively.
Theoretical and practical knowledge of Apache Beam can help you build you data stream pipelines in effective and efficient manner.
If you found this article insightful and want to sharp your knowledge further, I encourage you to explore my other articles on similar topics and other authors on Medium. Follow me for more in — depth tutorials, best practices, and real-world examples that will help you.
References
- Apache Beam Documentation: https://beam.apache.org/documentation/
2. “Streaming Systems: The What, Where, When, and How of Large-Scale Data Processing” by Tyler Akidau, Slava Chernyak, and Reuven Lax
3. “Streaming Data: Understanding the Real-Time Pipeline” by Andrew G. Psaltis
4. “Fraud Detection: Concepts, Strategies, and Real Applications” by Yongqiao Wang, Yongquan Lai, and Zhen Li
5. “Real-Time Analytics: Techniques to Analyze and Visualize Streaming Data” by Byron Ellis