Maven plugin development — from basic to advanced
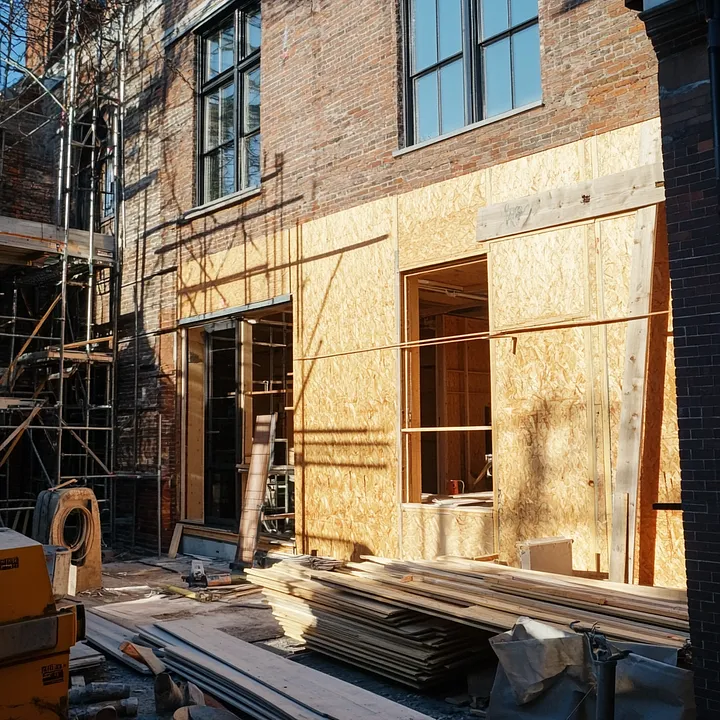
Introduction
Ever found yourself repeating the same mundane tasks during your build process? You might be checking forSNAPSHOT
dependencies before a release. You could also check code formatting or do custom verifications that regular plugins don’t handle. We developers love automation. Maven plugins help us do that. They let you tweak and customize your build process to match your project’s need.
In this article, I’ll guide you through creating your own Maven plugins, from simple to a sophisticated. After I missed SNAPSHOT
dependencies during a release and caused production delay, I saw that a custom Maven plugin could fix this issue for good. Why manually check when you can automate?
What is Maven plugin?
A Maven plugin is mainly a set of goals, also known as Mojos. These are Maven plain old Java objects that carry out specific tasks during the build lifecycle.
These plugins handle tasks such as:
- Compiling code.
- Running tests.
- Generating documentation.
- Deploying artifacts.
Here is how plugins fit into Maven’s architecture:
- Build lifecycle: Maven sets a standard build process. It includes phases like
validate
,compile
,test
,package
,install
, anddeploy
. - Plugins: plugin attach their goals to these lifecycle phases.
- Goals: Each goal performs a specific task and can be assigned to one or more phases or executed directly.
Some popular plugins are the maven-compiler-plugin
, used for compiling Java code, and the maven-surefire-plugin
, which run tests. You might know these example well.
Getting started with plugin development
Let’s start by creating a basic Maven plugin from scratch. We’ll use Maven’s archetype to generate the intial project structure.
mvn archetype:generate \
-DgroupId=io.vrnsky \
-DartifactId=maven-snapshot-detector \
-Dversion=1.0.0-SNAPSHOT \
-DarchetypeGroupId=org.apache.maven.archetypes \
-DarchetypeArtifactId=maven-archetype-mojo
This command creates a project with the basic stucture needed for plugin development. The generated project includes a simple Mojo (goal implementation) that we will customize.
Understanding the plugin structure
After running the archetype generator, you should have a project with this structure:
maven-snapshot-detector/
├── pom.xml
└── src/
└── main/
└── java/
└── io/
└── vrnsky/
└── MyMojo.java
Let’s take a look at the generated pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>io.vrnsky</groupId>
<artifactId>maven-snapshot-detector</artifactId>
<version>1.0.0-SNAPSHOT</version>
<packaging>maven-plugin</packaging>
<name>maven-snapshot-detector Maven Mojo</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>org.apache.maven</groupId>
<artifactId>maven-plugin-api</artifactId>
<version>2.0</version>
</dependency>
<dependency>
<groupId>org.apache.maven.plugin-tools</groupId>
<artifactId>maven-plugin-annotations</artifactId>
<version>3.2</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.apache.maven</groupId>
<artifactId>maven-project</artifactId>
<version>2.0.6</version>
</dependency>
</dependencies>
</project>
Notice the <packaging>maven-plugin</packaging>
element, which tells Maven this is plugin project. The dependenceis include maven-plugin-api
for basic plugin functionality and maven-plugin-annotations
for annotations like @Mojo
and @Parameter
.
Now let’s update the dependencies to use more recent version:
<dependencies>
<dependency>
<groupId>org.apache.maven</groupId>
<artifactId>maven-plugin-api</artifactId>
<version>3.6.3</version>
</dependency>
<dependency>
<groupId>org.apache.maven.plugin-tools</groupId>
<artifactId>maven-plugin-annotations</artifactId>
<version>3.6.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.apache.maven</groupId>
<artifactId>maven-project</artifactId>
<version>2.2.1</version>
</dependency>
</dependencies>
Creating your first plugin: SNAPSHOT
detector
Let’s create a practical plugin. This plugin will check for SNAPSHOT
dependencies in a project. It will help prevent accidental releases with unstable dependencies.
First, rename MyMojo.java
to SnapshotDetectorMojo.java
and update its content.
package io.vrnsky;
import org.apache.maven.model.Dependency;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import java.util.List;
import java.util.stream.Collectors;
@Mojo(name = "check-snapshots", defaultPhase = LifecyclePhase.VALIDATE)
public class SnapshotDetectorMojo extends AbstractMojo {
@Parameter(defaultValue = "${project}", required = true, readonly = true)
private MavenProject project;
@Parameter(property = "snapshotPostfix", defaultValue = "-SNAPSHOT")
private String snapshotPostfix;
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
List<Dependency> dependencies = project.getDependencies();
List<Dependency> snapshotDependencies = dependencies.stream()
.filter(dependency -> dependency.getVersion().endsWith(snapshotPostfix))
.collect(Collectors.toList());
if (!snapshotDependencies.isEmpty()) {
StringBuilder messageBuilder = new StringBuilder("Found snapshot dependencies:\n");
snapshotDependencies.forEach(dependency -> {
messageBuilder.append(String.format(" %s:%s has version %s\n",
dependency.getGroupId(),
dependency.getArtifactId(),
dependency.getVersion()));
});
throw new MojoFailureException(messageBuilder.toString());
} else {
getLog().info("No snapshot dependencies found. Your project is ready for release!");
}
}
}
Let’s break down this code:
- The
@Mojo
annotation defines the goal name (check-snapshots
) and the default phasevalidate
. - The
@Parameter
annotations inject Maven provided values: project — get the current MavenProject object.snapshotPostfix
dfines what string to look for (defaulting to-SNAPSHOT
). - The
execute()
method: 1. Get all dependencies from the project, 2. Filters for those with version ending in thesnapshotPostfix
, 3. If any snapshot dependencies are foudn, fails the build with detailed message.
Building and testing your plugin
Let’s build the plugin.
mvn clean install
This compiles the plugin and install it to your local Maven repository, making it available for testing in other projects.
Now, let’s create a test project to try our plugin.
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>io.vrnsky.test</groupId>
<artifactId>snapshot-test</artifactId>
<version>1.0.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>some-library</artifactId>
<version>1.2.3-SNAPSHOT</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>io.vrnsky</groupId>
<artifactId>maven-snapshot-detector</artifactId>
<version>1.0.0-SNAPSHOT</version>
<executions>
<execution>
<goals>
<goal>check-snapshots</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
When you run mvn validate
in this test project, the plugin will find the SNAPSHOT dependency. It should then fail the build and give an infromative message.
Advanced plugin development techniques
Now that we’ve created a basic plugin, let’s explore more advanced features.
Configurable parameters
We’re already added one configurable parameter (snapshotPostfix)
, but let’s expand on that concept. Parameters allow users to customize your plugin’s behavior. For example, we could add parameter to exclude certain dependencies from the check.
@Parameter(property = "excludePatterns")
private List<String> excludePatterns = new ArrayList<>();
// Then in the execute method:
List<Dependency> filteredDependencies = dependencies.stream()
.filter(dependency -> {
String id = dependency.getGroupId() + ":" + dependency.getArtifactId();
return excludePatterns.stream().noneMatch(id::matches);
})
.collect(Collectors.toList());
User can then configure the plugin:
<plugin>
<groupId>io.vrnsky</groupId>
<artifactId>maven-snapshot-detector</artifactId>
<version>1.0.0-SNAPSHOT</version>
<configuration>
<excludePatterns>
<excludePattern>internal\..*</excludePattern>
</excludePatterns>
</configuration>
</plugin>
Multiple goals in one plugin
A single plugin can contain multiple goals. Let’s add more relaxed goal that just warns about snapshots without failing.
@Mojo(name = "warn-snapshots", defaultPhase = LifecyclePhase.VALIDATE)
public class SnapshotWarnerMojo extends AbstractMojo {
@Parameter(defaultValue = "${project}", required = true, readonly = true)
private MavenProject project;
@Parameter(property = "snapshotPostfix", defaultValue = "-SNAPSHOT")
private String snapshotPostfix;
@Override
public void execute() throws MojoExecutionException {
List<Dependency> dependencies = project.getDependencies();
List<Dependency> snapshotDependencies = dependencies.stream()
.filter(dependency -> dependency.getVersion().endsWith(snapshotPostfix))
.collect(Collectors.toList());
if (!snapshotDependencies.isEmpty()) {
getLog().warn("WARNING: Found snapshot dependencies:");
snapshotDependencies.forEach(dependency -> {
getLog().warn(String.format(" %s:%s has version %s",
dependency.getGroupId(),
dependency.getArtifactId(),
dependency.getVersion()));
});
} else {
getLog().info("No snapshot dependencies found.");
}
}
}
Leveraging Maven’s tooling
Maven provides utilities that can be useful in your plugins. For example, you can access Maven’s settings, repository information, and more.
@Parameter(defaultValue = "${settings}", readonly = true)
private Settings settings;
@Component
private RepositorySystem repositorySystem;
@Parameter(defaultValue = "${repositorySystemSession}", readonly = true)
private RepositorySystemSession repositorySystemSession;
Creating an aggregator Mojo
If you want your plugin to run one for the entire project (rather than once per module in a multi — module project), you can create an aggregator Mojo.
@Mojo(name = "check-all-snapshots", defaultPhase = LifecyclePhase.VALIDATE, aggregator = true)
public class AggregateSnapshotDetectorMojo extends AbstractMojo {
@Parameter(defaultValue = "${reactorProjects}", required = true, readonly = true)
private List<MavenProject> reactorProjects;
// Implementation to check all modules
}
Unit testing your plugin
Comprehensive testing is essential to guarantee that your plugin functions as intended. Here’s basic test setup using JUnit and Mockito.
public class SnapshotDetectorMojoTest {
@Test
public void testNoSnapshotDependencies() throws Exception {
// Setup
SnapshotDetectorMojo mojo = new SnapshotDetectorMojo();
MavenProject project = createProjectWithDependencies(
createDependency("org.example", "lib1", "1.0.0"),
createDependency("org.example", "lib2", "2.0.0")
);
setVariableValueToObject(mojo, "project", project);
// Execute
mojo.execute();
// No exception should be thrown
}
@Test(expected = MojoFailureException.class)
public void testWithSnapshotDependencies() throws Exception {
// Setup
SnapshotDetectorMojo mojo = new SnapshotDetectorMojo();
MavenProject project = createProjectWithDependencies(
createDependency("org.example", "lib1", "1.0.0-SNAPSHOT")
);
setVariableValueToObject(mojo, "project", project);
// Execute - should throw MojoFailureException
mojo.execute();
}
private MavenProject createProjectWithDependencies(Dependency... dependencies) {
MavenProject project = new MavenProject();
project.setDependencies(Arrays.asList(dependencies));
return project;
}
private Dependency createDependency(String groupId, String artifactId, String version) {
Dependency dependency = new Dependency();
dependency.setGroupId(groupId);
dependency.setArtifactId(artifactId);
dependency.setVersion(version);
return dependency;
}
}
Publishing your plugin
Once you’ve developed and tested your plugin, you might want to share it with others. You have several options.
Local repository
The simplest approach is to install it to your local Maven repository.
mvn clean install
Company repository
If you’re working in a company, you can deploy to your internal Maven repository.
mvn deploy
make sure your pom.xml
has <distributionManagement>
section configured:
<distributionManagement>
<repository>
<id>internal-repo</id>
<name>Internal Repository</name>
<url>https://repo.example.com/releases</url>
</repository>
<snapshotRepository>
<id>internal-snapshots</id>
<name>Internal Snapshots</name>
<url>https://repo.example.com/snapshots</url>
</snapshotRepository>
</distributionManagement>
Maven Central
For wider distrubtion, you can publish to Maven Central. This process is more invlolved and requires:
- A group ID that you control (usually based on a domain you own).
- GPG singing of your artifacts.
- Javadoc and source JAR files.
- Maven metadata like SCM URLs, license information, etc.
Here’s a configuration example for Maven Central
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-source-plugin</artifactId>
<version>3.2.1</version>
<executions>
<execution>
<id>attach-sources</id>
<goals>
<goal>jar-no-fork</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-javadoc-plugin</artifactId>
<version>3.2.0</version>
<executions>
<execution>
<id>attach-javadocs</id>
<goals>
<goal>jar</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-gpg-plugin</artifactId>
<version>1.6</version>
<executions>
<execution>
<id>sign-artifacts</id>
<phase>verify</phase>
<goals>
<goal>sign</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Documentation
Good documentation is crucial for plugin adotpion. At minimum, include:
- A detailed README with purpose and benefits of the plugin, usage instructios, configurable options, examples.
- Comprehensive Javadoc for your Mojo classes and parameters
- Consider adding a site using Maven Site plugin.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-site-plugin</artifactId>
<version>3.9.1</version>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-plugin-plugin</artifactId>
<version>3.6.0</version>
</plugin>
Real — World example: A more comprehensive check
Let’s expand our snapshot detector into a more comprehensive release readiness checker:
@Mojo(name = "release-check", defaultPhase = LifecyclePhase.VALIDATE)
public class ReleaseReadinessMojo extends AbstractMojo {
@Parameter(defaultValue = "${project}", required = true, readonly = true)
private MavenProject project;
@Parameter(property = "checkSnapshots", defaultValue = "true")
private boolean checkSnapshots;
@Parameter(property = "checkTests", defaultValue = "true")
private boolean checkTests;
@Parameter(property = "checkScm", defaultValue = "true")
private boolean checkScm;
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
List<String> issues = new ArrayList<>();
if (checkSnapshots) {
issues.addAll(checkForSnapshotDependencies());
}
if (checkTests) {
issues.addAll(checkForSkippedTests());
}
if (checkScm) {
issues.addAll(checkForScmConnection());
}
if (!issues.isEmpty()) {
StringBuilder message = new StringBuilder("Your project is not ready for release:\n");
issues.forEach(issue -> message.append("- ").append(issue).append("\n"));
throw new MojoFailureException(message.toString());
}
getLog().info("Your project is ready for release! 🎉");
}
private List<String> checkForSnapshotDependencies() {
List<String> issues = new ArrayList<>();
List<Dependency> snapshotDeps = project.getDependencies().stream()
.filter(d -> d.getVersion().endsWith("-SNAPSHOT"))
.collect(Collectors.toList());
if (!snapshotDeps.isEmpty()) {
issues.add("Found " + snapshotDeps.size() + " SNAPSHOT dependencies");
}
return issues;
}
private List<String> checkForSkippedTests() {
List<String> issues = new ArrayList<>();
Properties props = project.getProperties();
if ("true".equals(props.getProperty("skipTests")) ||
"true".equals(props.getProperty("maven.test.skip"))) {
issues.add("Tests are being skipped");
}
return issues;
}
private List<String> checkForScmConnection() {
List<String> issues = new ArrayList<>();
if (project.getScm() == null ||
StringUtils.isBlank(project.getScm().getConnection())) {
issues.add("No SCM connection defined in POM");
}
return issues;
}
This expanded plugin checks seveeral aspects of related readiness:
- SNAPSHOT dependecies.
- Skipped tests.
- SCM connection details.
User can enable or disable each check through configuration.
Conclusion
Maven plugins are powerful tools for customizing and extending your build process. Create your own plugins to automate repetitive tasks. You can also enforce project standards and streamline your workflow.
We’ve covered the basics of creating Maven plugins. Set up the project structure. Define your goals. Configure the parameters. Then, release your plugin. We’ve also explored advanced techniques. These include creating aggregator mojos, leveraging Maven’s tools, and unit testing your plugins.
The example plugin we developed to detect SNAPSHOT
dependencies is just the tip of the iceberg.
You can create plugins to:
- Check your code formatting.
- Analyze dependencies.
- Generate documentation.
- Perform custom build tasks for your project.
Remember, effective plugins solve rela problems and make developers’ lives easier. If you keep doing the same manual step in your build process, think about using a Maven plugin to automate it.
References
1. Maven Plugin Development Guide
2. Maven Plugin API Documentation
3. Maven Plugin Annotations
4. Maven Plugin Testing Harness
5. Publishing to Maven Central