Kotlin features that makes me happy as Java Developer
Kotlin’s features liked by Java Developer
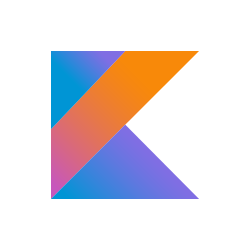
Introduction
The programming world continues to evolve, developer quite often seek languages that offer new one languages that are simple and powerful. Among modern JVM languages Kotlin has emerged as strong contender for those transitioning from Java. In this article I will highlight several Kotlin features that make me really happy
Type safety
Like a Java the Kotlin has static typing. Comparing with Java where nullability is often handled at runtime the Kotlin enforces strict nullability rules by default. It is means that variables must explicitly declare if the can be null this leads to reducing the risk of null pointer exceptions and making the code more predictable.
val a: String? //Can be null
val b: String //Cannot be null
Null safety
One of Kotlin’s most powerful feature for Java developers is its null safety mechanism. Kotlin automatically handles null values with a smart way to deal with them, allowing for safer coding practices without need for explicit null checks in many cases.
Conciseness
Syntax of Kotlin targeted to be concise and at the same time maintain clarity. This possible by following features:
- Property Accessors: Kotlin allows you to define properties with a single line of code, making the codebase more readable and easier to maintain
- Lambda Expression: The Kotlin’s lambda are shorter and expressive than in Java
Functional Programming Support
Kotlin integrates functional programmings concepts into its syntax, allowing developers to write concise and expressive code without the need for external libraries or framework. Features:
- High-order functions: Kotlin supports higher-order functions that can take other functions as arguments or return them as result
- Lazy evaluation: Kotlin’s lazy properties allow for efficient resource management by delaying computation unit it is absolutely necessary
Interoperability with Java
Kotlin is designed to interoperate with Java, making transition smooth for developers familiar with the Java ecosystem. This includes
- A lot of common libraries and frameworks: Kotlin can use the same libraries and framwroks as Java
- Java interoperability: Kotlin code can mix with Java code without requiring any special annotations or configurations
Safety in Concurrency
Kotlin provides built-in support for concrenncy with:
- Suspend functions: Kotlin’s coroutines make developers able to write asynchronous code that easier to understand and maintain than traditional callbacks
- Concurrent collections: Kotlin offers a lot of optimized concurrent collections well suited for multi-thread environment
Modern Language Features
Kotlin incroporates modern language features like:
- Default parameters: By this you can define functions with default values for argument. This makes your code more flexible and easier to use
- Extension functions: Kotlin enables adding functionality to existing classes without modifying their source code, promoting a clean separation of concerns.
Example of default parameters. The below listing demonstrates how developers can set default value for separator argument
fun split(input: String, separator: String = ", "): List<String> {
return input.split(separator)
}
For example you have following data class in Kotlin
package vrnsky.io.model
import kotlinx.serialization.Serializable
import vrnsky.io.LocalDateSerializer
import java.time.LocalDate
@Serializable
data class Subscription(
var id: String?,
@Serializable(with = LocalDateSerializer::class) val startDate: LocalDate,
@Serializable(with = LocalDateSerializer::class) val endDate: LocalDate,
)
You want to add some functionality without modifying it. The extension functions coming to help you. The example of extension function below
package vrnsky.io.extension
import vrnsky.io.model.Subscription
fun Subscription.withId(id: String): Subscription = Subscription (
id = id,
startDate = endDate,
endDate = endDate
)
Conclusion
A lot of great features provided by Kotlin such as type safety, null safety, interoperability with Java, concurrency management. This all features make Kotlin attractive choice for Java developers looking to enhance productivity and write safer, more maintable code.